Deploying next js app is quite simple yet quite tricky. When I was deploying my first next js app on ubuntu server I didn't find much tutorials. So I decided to write this article which might be helpful.
You can follow this tutorial if you want to deploy your next js app on your private server i.e. vps (eg: digitalocean server) having ubuntu as an operating system.
Step 1: Login to your vps server using given credentials from service provider
ssh USERNAME@IP_ADDRESS // Eg: ssh root@your_ip_address
Step 2: Install nginx, yarn and pm2 on your server globally.
// Install nginx
sudo apt install nginx
// Install yarn
curl -sS https://dl.yarnpkg.com/debian/pubkey.gpg | sudo apt-key add -
echo "deb https://dl.yarnpkg.com/debian/ stable main" | sudo tee /etc/apt/sources.list.d/yarn.list
sudo apt-get update && sudo apt-get install yarn
// Install pm2
yarn global add pm2
Step 3: Fetch your next js app from github and install dependencies
git clone GITHUB_PROJECT_URL
cd project
yarn install
Step 4: Set build directory and run yarn build to run your app on production
Next js serves the requests from single build file which is inside ./next directory by default. You can keep it as it is but sometimes it doesn't serve static files properly on custom server so I recommend to create build directory inside project root by ading next.config.js file and paste the following code inside this file.
module.exports = { distDir: "build" };
Now run 'yarn build' inside project directory and make sure there is a build folder with all of your project code bundled at one place.
Step 5: Run project using pm2
Run following command inside project directory to run your project.
pm2 start yarn --name "myapp" --interpreter bash -- start
Now your app must be running at http://localhost:3000. The port number could be anything you have set on your application.
Step 6: Connect localhost to server using nginx.
Go to nginx config file using following command
sudo nano /etc/nginx/sites-available/myapp
Then replace the content with following code:
server {
server_name _;
location /build/ {
# This is the full path of your project build
alias /home/username/project_directory/build/;
expires 30d;
access_log on;
}
# The port must be exactly the same with your app port i.e. 3000 for our case.
location / {
# reverse proxy for next server
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Make sure your nginx configuration has no syntax error
sudo nginx -t
Then restart nginx
sudo systemctl restart nginx
Step 7: Check you server IP (Public IP or domain name) on browser, your app must be live.
Furthermore, you can update you app if new code is updated as follows.
# Go to project directory
git pull origin master
yarn install
yarn build
pm2 restart myapp
Congratulations! You now have your next js application running behind an Nginx reverse proxy on an Ubuntu 18.04 server.
Binod Chaudhary
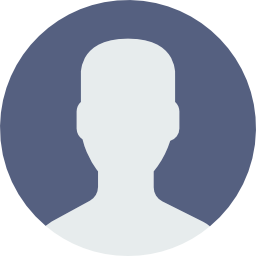
Software Engineer | Full-Stack Developer